We had a course in programming. It was just basics, would say directed to those who don´t have any experience in coding. Java was used and mostly went thru arrays, loops and object oriented. Anyhow, the assignment was two parts, one to write a Morse code project, two written report.
I wrote a small tutorial just to show backend – frontend connection and how a project might be set up. I will post that later on, since the feedback I got from my classmates was that it made it easier for them to understand. But ack to the course assignment.
The written assignment requirements:
- Describe the following concepts within Java:
- Dot notation
- Return
- Array
- Exception
- Describe a Data structure
- Describe TDD, Test Driven Development
- Describe your choices of project structure in the code project
- Give two example
Describe the following concepts within Java
Dot notation
Is used to access a class or a member of a package. Sometimes also called member operator.
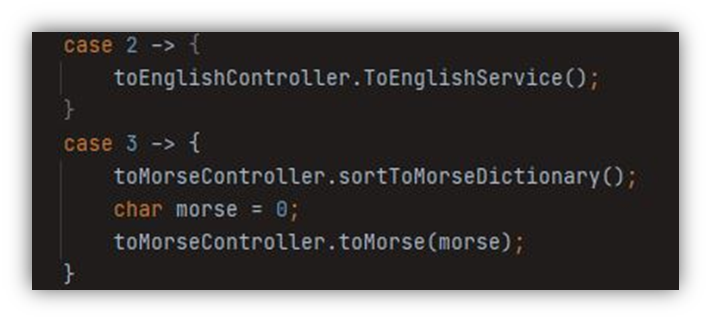
In the example above we have a toMorseController Class with a sortToMorseDictionary method. And a toMorse method with the object morse.
In Object Oriented Programming each object is an instance of a class or a type of object. And dot notation like the example above allows us to tell a instance of a class that we want to use a method inside of the class.
Return
The return keyword is simply explained the exit of a method. If the method is declared void, we don´t need to declare a return statement since it does not return a value. Can be left without Return or be written as empty Return; If a method is not declared void, we need to include a return statement, this must include a value that match the methods declared return type. And the return statement needs to be declared inside the body of the method.
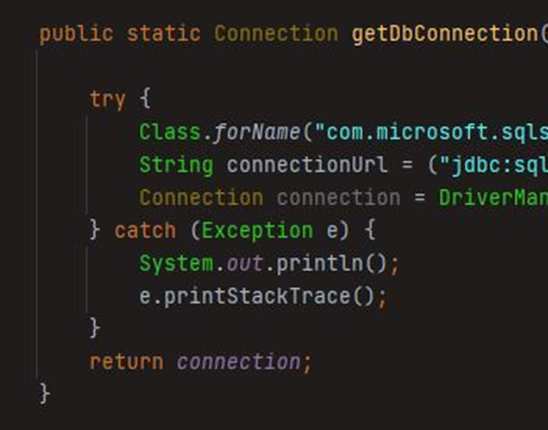
Here we have a Connection method that makes the value of the return statement to be of the Connection type.
Array
Instead of declaring a variable of every value we need to manage we can declare one that includes a collection of them all. So, to say a container collection that holds fixed numbers of values. The values must be of the same type. The elements in the Array are accessed by its index, index starts counting on 0. It is one of the most common data structures used. We use length to find the total number of elements (objects)in an Array.
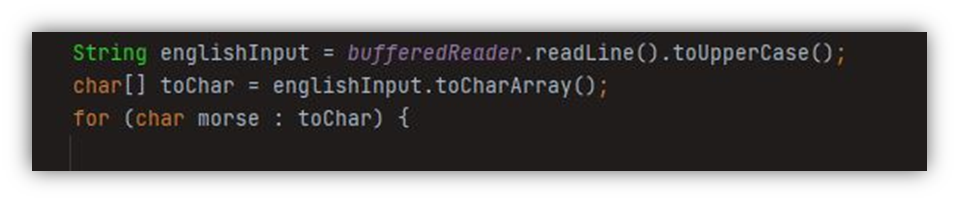
In the code above a char Array is used. It holds character data types values.
Exception
An event that during the execution of a program will tell us if there are any disruptions. There are several types of exceptions. We can put them in two categories: Compile Time Errors and Run Time Errors.
- Compile time errors are errors caused by incorrect syntax, which prevents the code from running.
- Run time errors occurs while the program is running.
- Exceptions can occur at both and can be checked exceptions or unchecked exceptions.
- Checked Exceptions are checked during runtime and unchecked are checked at compile time.
Two examples of checked exceptions are Try-Catch block, or we can use the throws keyword. Throws keyword is used to declare an exception and gives us information that there may be an exception of the listed types of exceptions. We can declare multiple exceptions at once with the throws keyword, this can make the throws keyword more convenient to use in cases where we have methods that might need to manage several of different exceptions. It keeps the code clean compared to writing several different try-catch blocks.
Data Structure
To store and organize our data in an efficient way we can use data structures. This can help us reduce the time and space of different tasks.
Some examples of Data Structures:
- Arrays
- Linked Lists
- Queues
- Trees
- Stacks
- Hashing
- Graphs
One example of Data Structure is HashMap. It is used to store mappings of key-value pairs. There are some rules about the use of HashMaps: Only one key can have null, but the value objects can have multiple. We can not use primitive keys or values. Keys must be unique because it uses hashing to calculate index and is applied onto the key object.
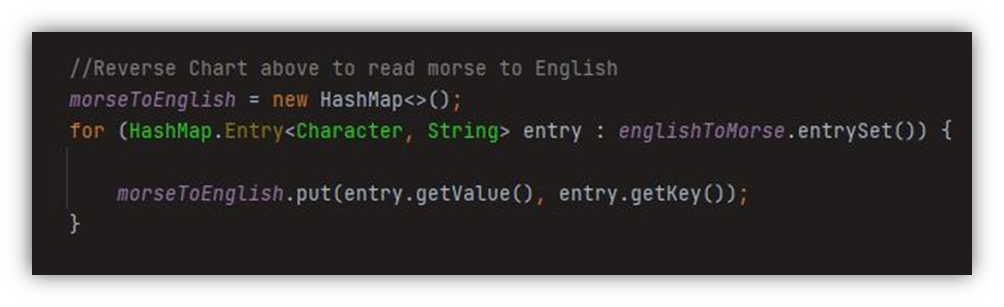
In the example above we have a method that revers a Morse code dictionary to read morse to English. The entrySet() method is used to create a set from the same elements we have in the HashMap above. From this we can create a new set and store the map elements with getKey() and getValue().
Development with TDD
Test Driven Development (TDD) is a development process or if you want a technique in which test cases are developed before a feature implementation. Based upon requirement conditions we write test cases for each function to check the feature.
The process is basically a step by step based on unit tests:
Write test. Run test with a failure as expected result. Update and correct the code to make it functional. Run same test again and it should pass as successful test. Refactor and repeat.
This way of development makes it easy to use it as a design technique, we keep focus on each small method of the code. Less risk of exceptions, less duplication of code, easy to follow the run thru of methods and it makes the code pretty much self-documented. While we use mostly use Don’t Repeat Yourself, DRY principle for our production code, we can use Descriptive and Meaningful Phrases, DAMP for the test code. It of course depends upon project and its factors but could be an effective way to make our unit tests be our documentation. A way to easier see what and what not to avoid exceptions in our production code, when implementing after test cases are written.
Code Project Report
For our Morse Code project, I choose to use HashMap for the Morse Code dictionary. The reason for this is the efficiency of this Data structure. It is a straightforward way to store the key and value for both char and string. With this we can easily revers the Map between English to Morse and Morse to English. (as in the section above about data structures).
It is few classes needed for this small project, but I still used the design of MVC to make the project structure clean and the code self-explained. The view class keeps only the code for the console view, and the logic is written in the controller class. Since we use Object Oriented Programming, we model the use of our objects.
The exception handling used is Throws and Try-Catch blocks and of course the default used in the menu switch case.
I also added a menu feature to see a list of the English Morse Code Dictionary. This to make it easier for the user to double check the dictionary, since morse code might not be our first typing language… Here I wanted to sort the Morse Code list and used the Map Interface or more specific the NavigableMap Interface and AbstractMap class. TreeMap class implements those two. It stores key-value pairs in a sorted order, by sorting the entries according to their natural order. And holds only unique elements.

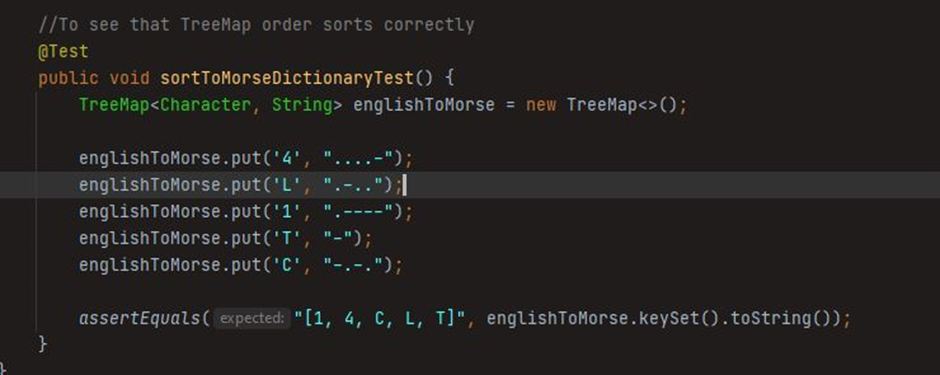
That was done with the method above.in the first photo above.
And to make sure it was working the way I wanted a test was written before the method.
Summary
A summary of things used in the Morse Code project:
- MVC
- TDD
- OOP
- Java
- JUnit
- Hamcrest
- Exception handlers
- Throws
- Try-Catch blocks
- Switch case Default
- Data Structures
- Array
- Char Array
- HashMap
- TreeMap
The Morse code project can be found on my GitHub page: Link to project
Latest Posts – Testing