The goal with the project is to build a sitemap generator, where you type in your domain and it generates XML sitemap that you can download. There will be a free version and a subscription version. If you subscribe you will have access to more features, like save domains, set an automatic crawl calendar etc. Since the extra features are available only by subscription, we need to build a log in and payment system.
The purpose with the project is to learn using PHP and the framework Laravel. To see how you can build web applications by combine readymade package libraries with self-written functions. The project is supposed to give an understanding how Laravel’s structure is built up by using MVC. And also, how you build a payment service using Laravel Cashier. The project will be using Bootstrap for layout and design will be made by frontend-developers.
The public index page:
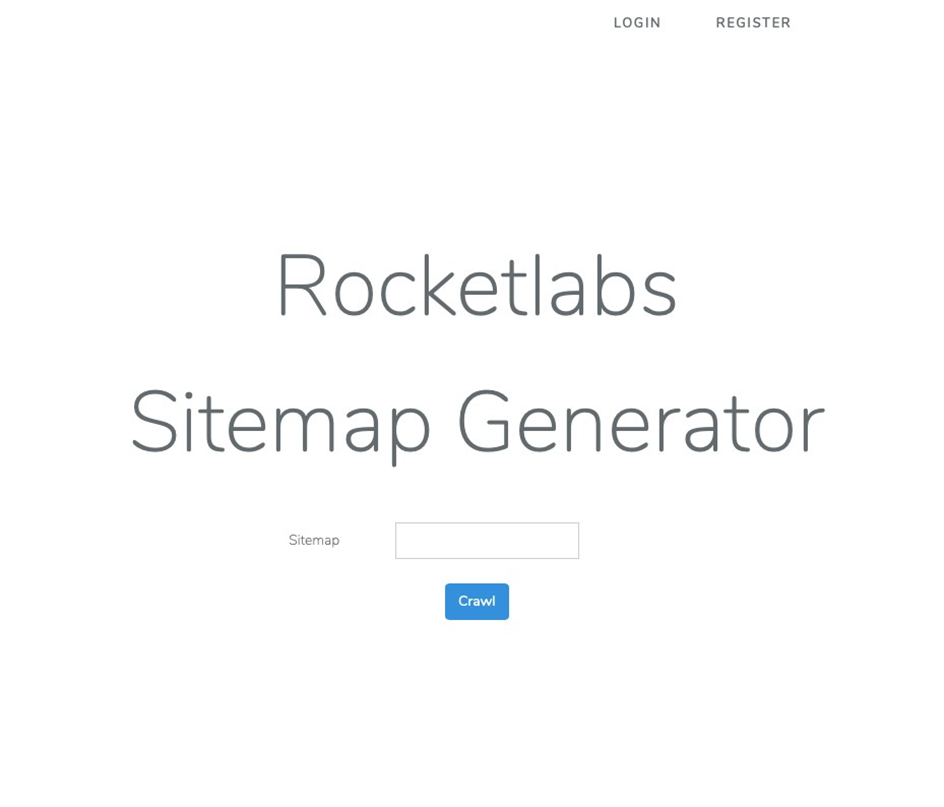
If the person wants more features and to be able to save projects and update by setting a schedule for generating new sitemaps, they can create an account and subscribe to the service.
Starting the main project, team planning
We did have a short daily scrum/ stand up the first weeks. Just a short meeting where all of us said what we had done, was going to do. As mentioned above, we had pretty much free hand how to do the code, in the project description some features were set and that was what we were working towards.
The account and subscription part of the site was going to have some specific features. And the different services you can subscribe to was only going to be shown if you had subscribed and your payment is complete. When creating an account, you will get 2weeks trial time. This information needs to be stored in the sites database. After that you need to subscribe with a bankcard.
Since the site itself is not handling any payment details we had to use a payment service, Stripe. And the site was going to have multiple language support, in Swedish and English.
So, to be able to create this project with these specific features we need to use some different framework, platforms and packages.
Laravel, Laravel Cashier, Laravel Localization, Stripe
And also:
Laravel Link-checker, Laravel Sitemap, IDE: PhpStorm, Composer, Php Artisan, Xampp: Apache, MySQL, ProFTPD, Homebrew, Ngrok, Code pattern: MVC
Knowledge and new learning:
There are some things in this project that I´m already familiar with, like MVC, SQL and routing is something we have been using in .NET core courses. Homebrew, Ngrok I have been using before in my own home projects, working in Kotlin, Xamarin & C#.
So, with those things being familiar it was great fun to jump in to learning all the other new features.
Writing the functions for account and subscription:
Usage of different packages and platforms:
When you start a new Laravel Cashier project it will generate a readymade Login / register set up. But in this project, we are going to use Stripe too and the only feature (except the payment details and card handling) that is not connected to both database and Stripe is the start of the trial time, that will start directly when a user creates an account. So, the first thing was to create the connection between the database and Stripe.
That also included to create the price plans, so we can keep track of what plan the user chosen, if payment is complete, when trial ends and so on.
Create subscription plan on Stripe:
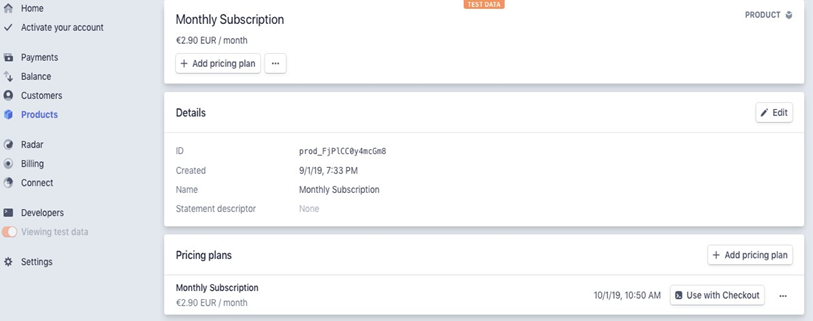
Create Subscription plan in database:
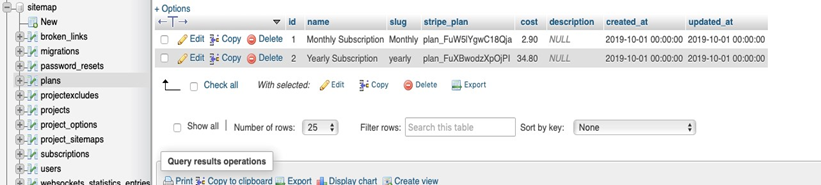
The database is setup thru Xampp, so it´s easy to use and make the connections needed. The database we have also included all the tables seen on the left side of the picture. The ones set up with the user and payment system is user and subscriptions.
Now we have all that set up, so next step is to create the payment system. Here we will mostly use stripe and its features. The reason for this is that we don´t want to store the sensitive information from users in the database, the only thing that can be seen in the database is the last four digits of the card number and card brand. The rest will be handled by Stripe.
Stripe
And what is Stripe? It is a payment platform including applications to manage revenue, prevent fraud, and expand internationally. It is possible to let Stripe handle everything from using their templates and readymade code for all of the steps in a payment chain.
But since we are using Laravel Cashier we will be writing some of these things in the project. And writing code is of course the fun part.
There were a few things that challenged the start with Stripe for me, first I have never used either of Stripe or Laravel, this made it bit difficult to sort out which functions/methods that was needed to be coded from the different ones. Like when reading Stripes documents they of course explain mostly how to use their services in all features. And Cashier has some smart readymade code that simplifies the connection to stripe. It saves time to use Cashiers methods/functions in many cases where it is available. But more about that below. Another thing that made it important to really understand the set up for the subscription payments and authentication, is the new regulations in Europe.
As Stripe describes it on their site:
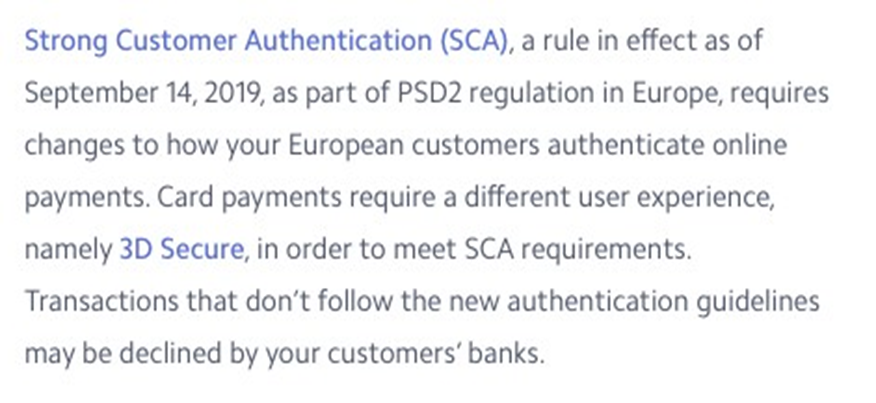
This was set to start just 2 weeks into our learning in work environment time, so brand-new rules. Took some time to figure out the rules and what was needed to get it set up correctly to not cause problems in the future.
To use stripes features in our project the code used is HTML in the blade.php files (view files) and also JavaScript. Both of these I have used before, so it felt familiar working with. Also, very good to get an update in how to write that sort of code. Felt like I learned some more there too.
When handling card payments there are a couple of different scenarios that needs to be handled, like incomplete payments, expired cards, frauds, taxes, calculation of invoices. And the smooth thing here is that Stripe does all this, no worries about those parts on our side. All events can be tested easily thru webhooks, using Ngrok connection.
And speaking of webhooks, to send our requests back-and-forth between Stripe and our site we are as mentioned going to use Laravel Cashier.
Laravel Cashier
Laravel Cashier is a ready-to-use package. It provides an interface for managing billing services provided by Stripe. It handles most things like swapping subscriptions, trial periods, send invoices as PDFs etc.
To set up Cashier and understand their guide was the most challenging part of using Cashier. A couple of things was the reason for that. Of course, the fact that I haven’t used PHP at all before made it all new. But I think the logic behind the code language is pretty easy to understand, it isn´t that far away from other code I have been using before. What was more so challenging was to understand the way the guide is written; it is different to most documents about for example C# & .NET Core. Way more minimalistic, it gives space for misunderstandings and guessing’s when you isn´t familiar with the set-up of the framework. And also, when facing problems on the way there is not much other documentation to find that is correct at the moment. This also because of the new SCA rules, Cashier had a big update just around the days we started the project. Although I have to say that it was a great fun thing to get started to read up and learn this, all new and inspiring. In the start I was missing some logic that .NET core has and that is more familiar to me, but along the way I got to appreciate Laravel´s really well thought readymade features.
To show how it might look, I mentioned in the start that when a user registers an account on the site the user will get 14 days free trial at first, without payment method upfront. But to continue using the service they need to subscribe. That means there has to be a user account created on Stripe too, and to do this and lots of other functions to connect Stripe, Cashier gives us functions to do so out of the box:
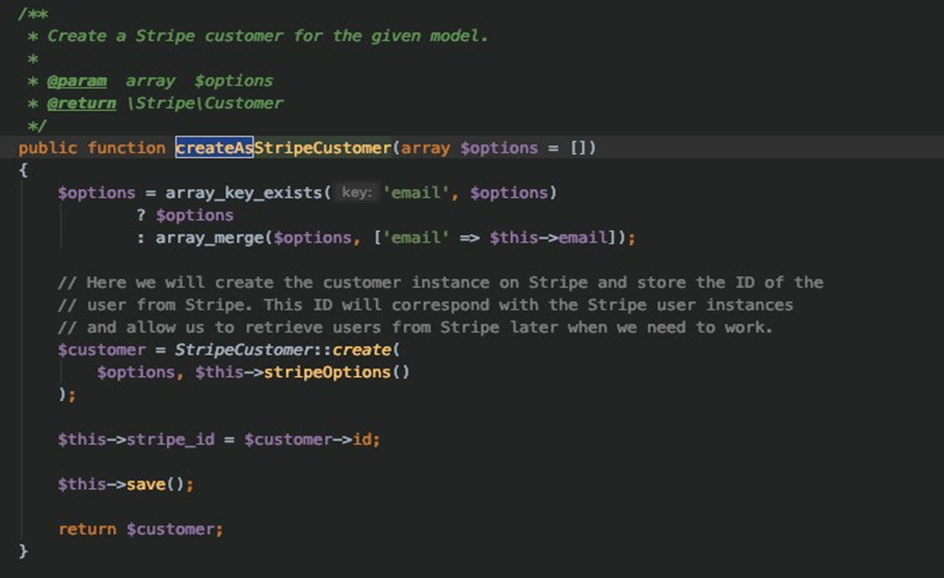
Other things that needed to be handle in the project is as I mentioned, to see if the user has incomplete payment, if the trial has ended, to give the option to cancel subscription, to delete account and more.
All these things are set up in similar ways. Used an MVC pattern for the code. Another thing is Cashier has a function for is to give the user the option to download invoices, and if the user changes subscription plan, Stripe does the calculation of the amount automatically for us, smooth!
Here is how the invoice that comes with Cashier looks like:
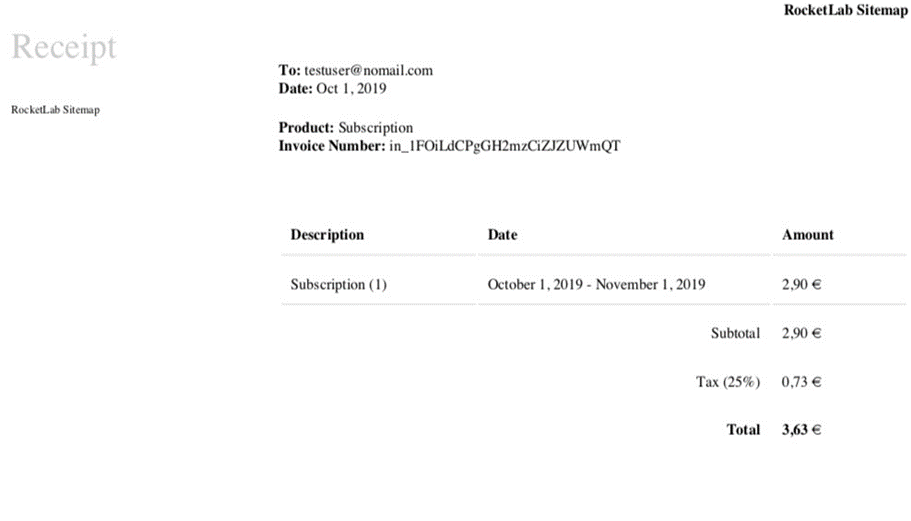
Laravel Localization
Another feature that we were going to add to the project was language control, the option for a user to select between English and Swedish.
This was made using Laravel Localization, another Laravel package that provides a convenient way to retrieve strings in various languages. Here I didn´t do much work more than setting the features up and do the routing for it to work on all pages. Even if I didn´t go into depth in the understanding of it all, it was another fun thing to learn more about. The rest of the work, to actually make the feature visible in a nice layout to the user and translation of the texts is on the Front-end-side of the project.
How PhpStorm looks like, not so different from like Visual studio:
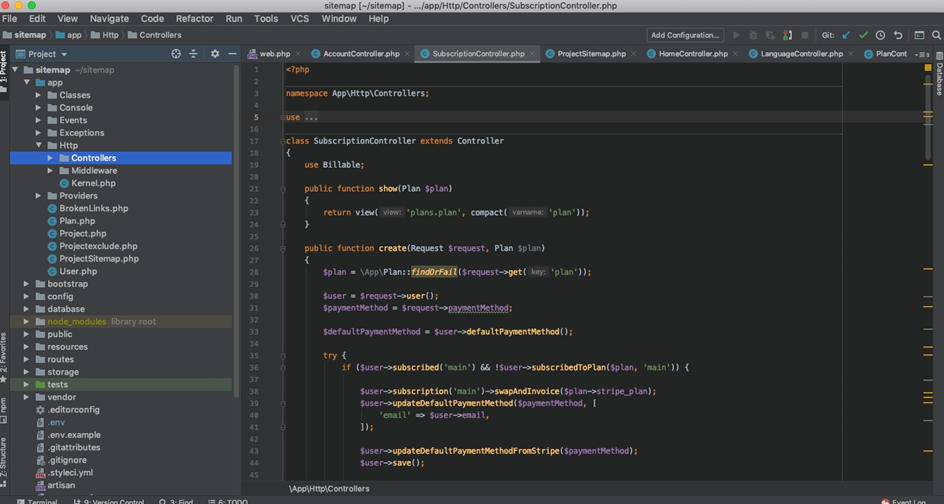
And how it looked before the frontend started their work on the deisgn:
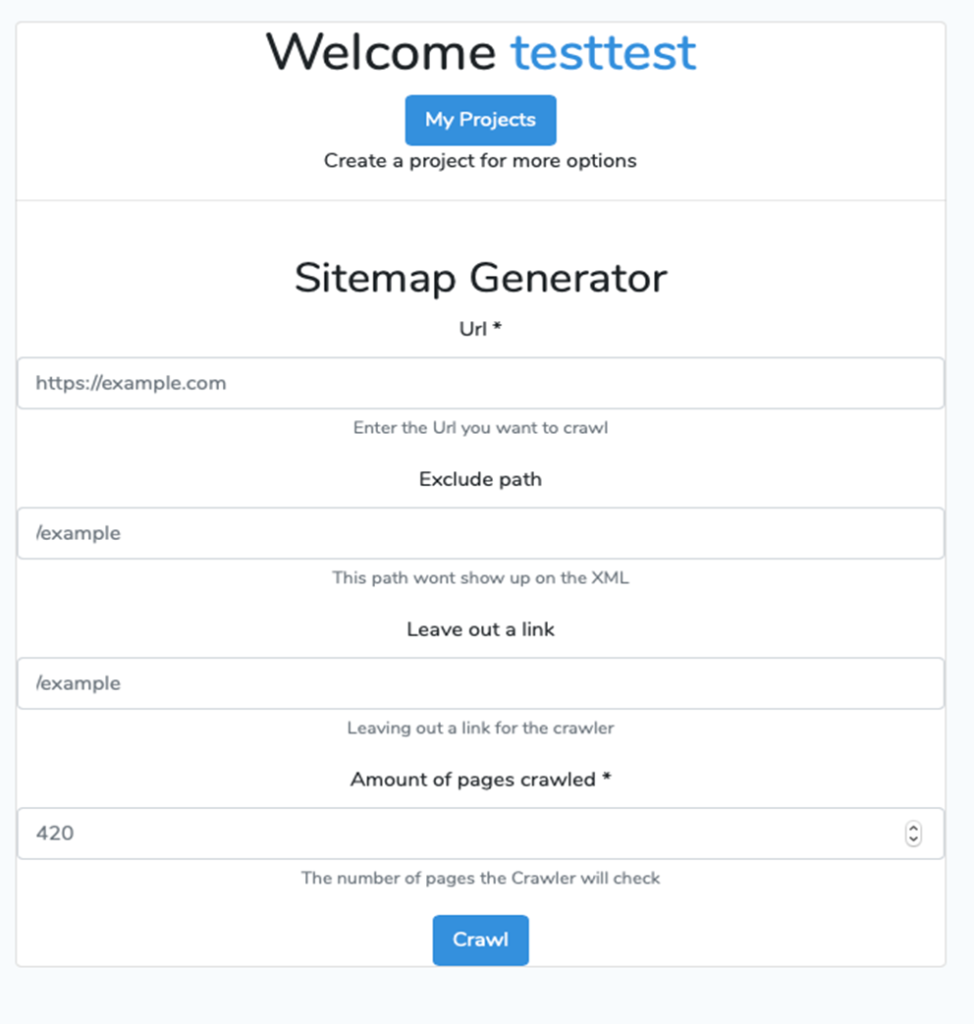
Ok, I have to admit that at first working in PHP wasn´t something it had a big interest in. Not negative about it but not really inspired either. And these weeks has been way over my expectations. The project we got and the way it was set up was really something that suited me and made me feel comfortable to try set up the code and write it from the knowledge we got and also to try new things. I really felt like I learned a lot and got to improve areas I was insecure about before. And my thoughts about PHP now? With Laravel Framework, it is definitely something I can work more with.